What is Service Worker?
It is an individual JavaScript file, which runs in a separate JavaScript thread in the browser, for a specific domain, specific path, intercepts and manage network calls, and provide features like background sync, push notification etc.
Service Worker will be always active for a website, even if no tab opened for the website. So, it gives seamless push notification and background sync experience.
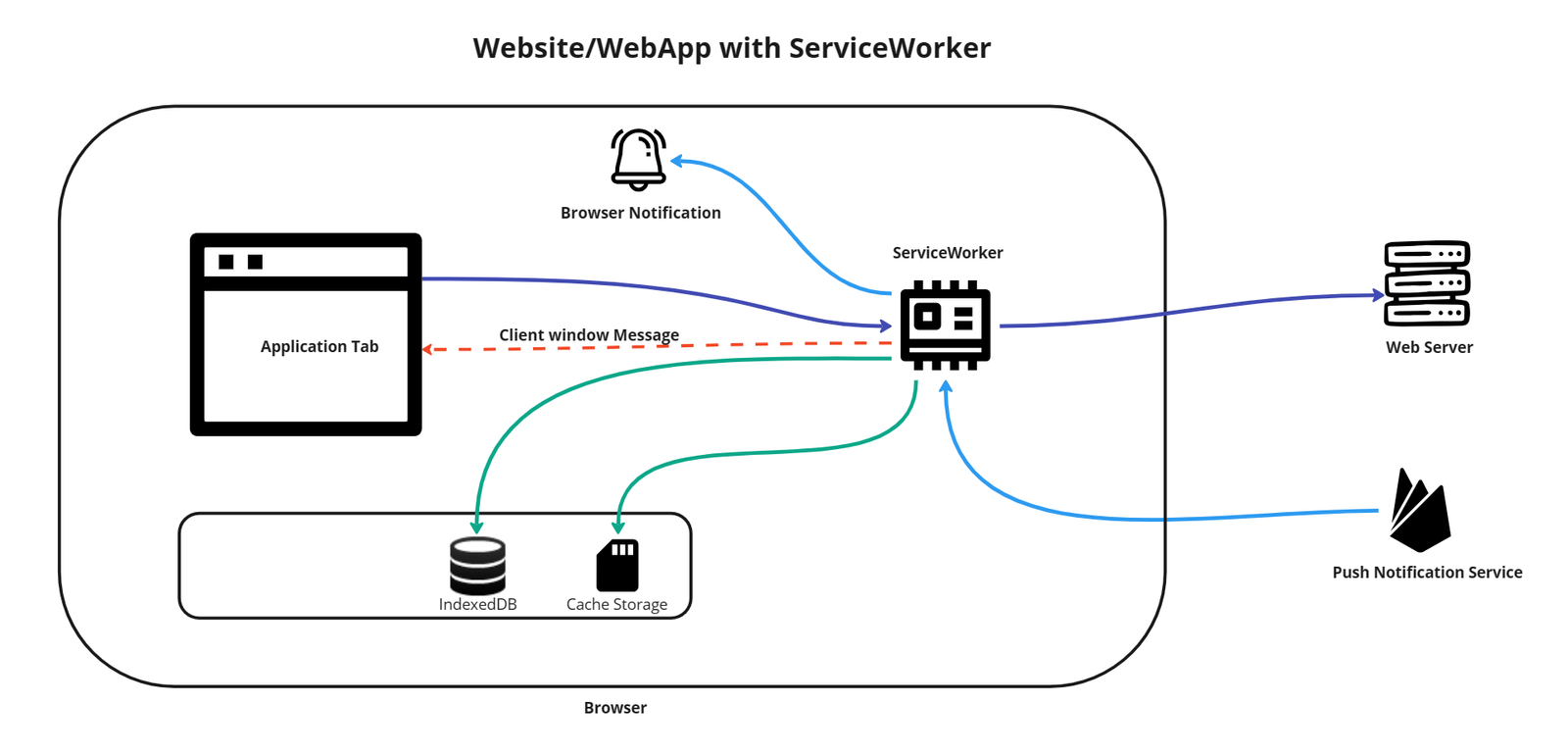
What can we get from Service Worker?
- Offline Capable PWA
- Push Notification integration
- Faster Static resource loading for frequently visited websites/WebApp with/without CDN
- Control on Network requests
The Common use case for using Service Worker in your web application is – when you are going mobile first (as 90% traffic is expected from handheld devices), you need lightning first web pages. Service Worker can help you to build progressive cache, so user never feels lag in screen switching, instant results and partial static resource rollout to the device.
Common queries & confusions about Service Worker
What framework should I prefer to get support of Service Worker?
Service Worker does not care about what framework you are using to develop your Web App. You can use with React, Angular, Vue, Native JavaScript .. anything. Infact, most of the frameworks & package builders like CRA, Vue CLI, webpack, Vite etc provides templates to generate application base with Service Worker /PWA support.
Can I implement Service Worker in existing project?
Yes, you can implement service worker at any point of time with your application. As Service Worker is an individual file and controls the network traffic by playing role of kind of proxy client – so there is no issue at all. Even you can use Service Worker with MPA, SSR or SPA any type of projects.
I have deployed Micro-frontend Applications in same domain, only paths are different, Can I use Service worker?
Yes, practically you can have individual service worker running in different path for different micro frontend application. Cache Storage can be manged with registered path (so no confusion on cache management).
My application is hosted in a path instead of direct root of the FQDN. Can I run Service Worker on root directory level?
Yes, you can register your service worker on root level, even though your application is running in a child path using registration scope. you can check the documentation on MDN.
As Service Worker is working like client Proxy, So, can I handle POST API calls?
Yes, you can control POST or any other methods using service worker. For example, if some operations are failing for some temporary reasons, you can make entry on indexedDB and using Service Worker, you can try to execute the queued actions periodically. Google Analytics heavily uses analytics event queue.
My Web Application is deployed in 4 Web Servers, can I update Service Worker file directly by logging into the server?
Never ever try to update Service Worker file manually in the servers. As the creation/modification time/Character/Space will be different, every time browser will reach a random server and the Service Worker File information mismatch will happen and your browser will reinstall the Worker every time. So, better go via build and deployment process to keep the information same.
Can I change my Service Worker File name?
Practically Yes, but not recommended and dangerous. As the service worker file is getting installed into the browser, and there is a proper lifecycle of every Service Worker, then you have to make sure that the previous service worker is uninstalled with its cache scope and then only the new Service Worker is going to install. For this, you might be ending up with multiple deployments.
Where will Service worker not work?
When your app is not running on valid HTTPS enabled FQDN, or your App is getting accessed via IP only, Service Worker will not work. On localhost it will work anyway for debug purpose – try to run the application on port 80.
Technical Understanding of Service Worker
Lifecycle
- Registration – This is the only area which get initiated from your application code base. You provide the Service Worker file name for registration/unregister.
- Download & Installation – From this point, all the life cycle events happen in your Browser, and you can check in Application Tab
- Wait – Service worker by default does not get activated immediately in same session, it waits for the active tab to get closed. You can utilize Skipwaiting to activate the Service Worker ASAP.
- Activation – In this stage, it will do all required/mentioned operations (like downloading files, replace the old cache), it is getting ready to take control on next launch
- Activated – This state indicates that the Service worker is in action / controlling network traffic.
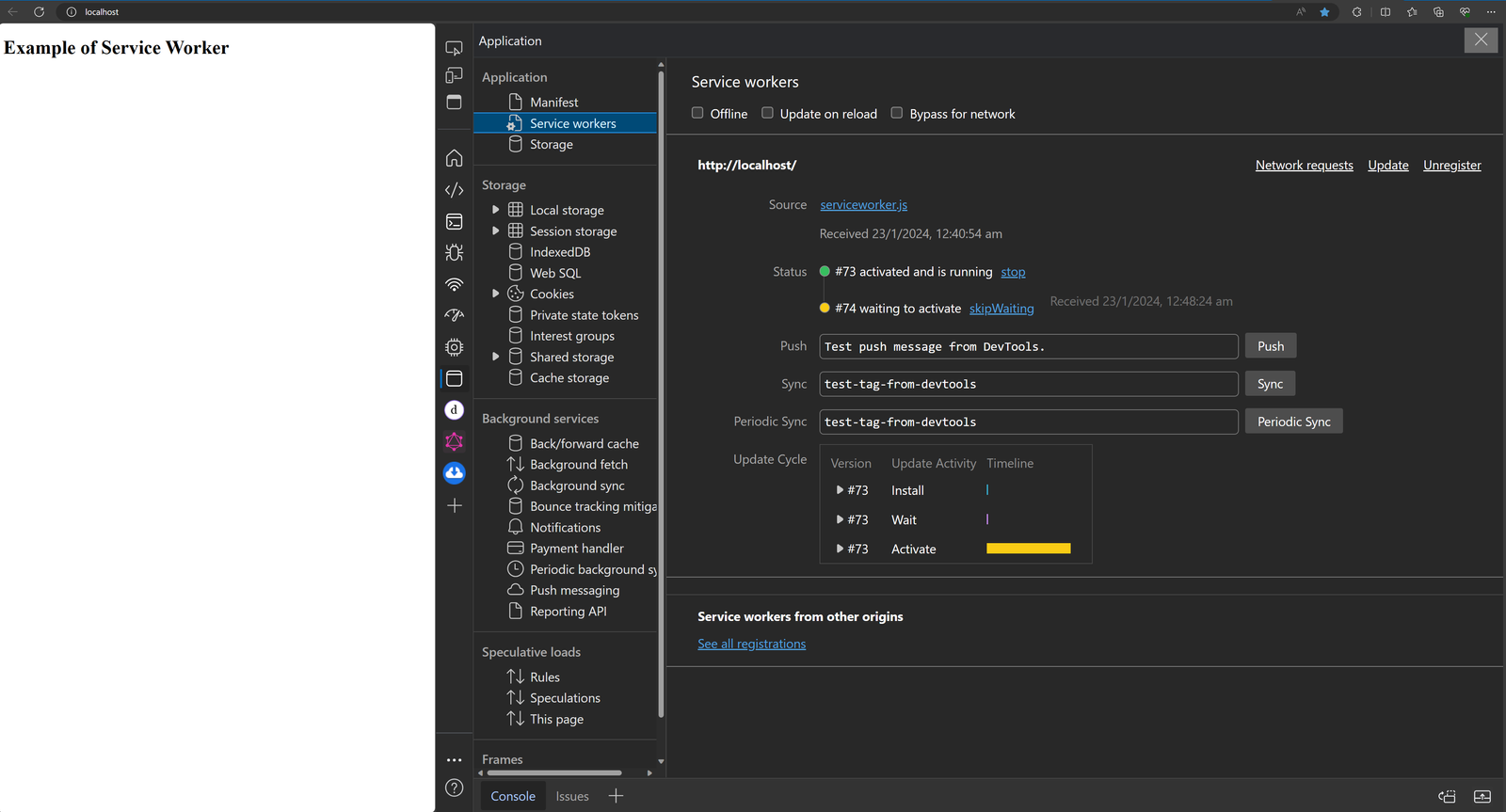
You can get more detailed documentation on MDN Service Worker Documentation.
Events
- Fetch – Whenever any network calls made from browser to network, this event will be triggered, here you can take control of the network call and you can apply your response strategies like – Cache First, Cache Only, Network First, Network Only etc.
- Sync – Whenever the Service worker File sync will happen with the server, this event will be triggered (time to time it will check for update whenever you have good network connectivity).
- Push – Whenever any Push communication received, this event will be triggered.
- message – You can capture text messages sent from webpage using window messaging. Similarly, for active clients you can send messages.
Common Keywords & Methods
- self – refers to Service Worker Global Scope
- client/clients – Open instances/active Tab(s) on same application – you can take control, or send message to them
- skipWaiting – Skip the wait state to activate immediately.
- caches – Access the Cache Storage to play with request/response cache
- indexedDB – Access the IndexedDB instance for the FQDN
- location – location object in context of the Service Worker. Don’t mix up with webpage location.
- importScripts – You can create the service worker in distributed structure and include them using importScripts method.
- registration – You get access to many features like push manager, cookies etc.
Library references for Service Worker
The best available plugin/library is Google Workbox.
You will get help with blacklist/whitelist URLs (where service worker network control will work or not). Ready to use cache Strategies. Google Analytics offline Sync. full PWA support.
You might face challenge with query parameters in URL, POST and similar method control. You must write your own logic to handle these scenarios.
For Strategies you may refer below links –
Strategies for service worker caching | Workbox | Chrome for Developers
mdn/serviceworker-cookbook: It’s online. It’s offline. It’s a Service Worker! (github.com)